Category Archives: Source Code
Modulo operation and circular array
Combinations of adding valid parentheses
Build hierarchy tree
Josephus problem using circular linked list
Topological sort using DFS and BFS
Word ladder using bidirectional BFS
Autocorrect and edit distance problem in Java
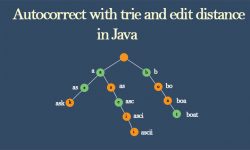
When you search in Google, it provides autocorrect for validating the keywords you enter into the input box. Behind the scene, it checks the input words against a dictionary. If it doesn’t find the keyword in the dictionary, it suggests a most likely replacement. Here I introduce a simple solution …
Domino Eulerian path problem using backtracking
Permutation of multiple arrays and iterator – code
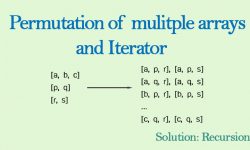
Permutation of multiple arrays and iterator has two tasks. First is the permutation of multiple arrays and output as an array of new combinations. The second is to implement the iterator of this new array. Permutation is an important topic in the fields of combinatorics. It is usually implemented using …